I was recently tasked to build a script to logoff a group of sessions from a CSV file, which contains usernames. The script requires PowerCLI and HV.Helper modules, like most Horizon scripts. Also note that this will only work in a Cloud Pod Architecture environment and will actually logoff sessions across all pods. I can add the capability for a single / localized pod in a future version as well, if needed/requested.
There are two arguments here – CS (Connection Server) and CSV (path to the CSV file, or name if already in current working directory).
Please note that the format of the CSV file must contain username as the header and FQDN\username format, such as vmware.com\nick:
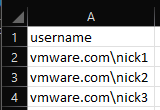
# CSV MUST have username header and FQDN\username format for usernames, such as vmware.com\username
param (
[string]$CS,
[string]$CSV
)
#####################
try
{
Connect-HVServer $CS
}
catch
{
Write-Host -ForegroundColor Red "Login seems to have failed! Make sure to use DOMAIN\username format and that you have appropriate PS modules!"
Read-Host "Press enter to continue..."
exit
}
# populate usernames from CSV
try {
$usernames = import-csv $CSV
Write-Host "There are"$usernames.count"usernames in the CSV!"
}
catch {
Write-Host "Failed to import CSV! Ensure path is correct"
exit
}
# populate API extensions
$HVServices= $global:defaulthvservers.ExtensionData
$sessions = Get-HVGlobalSession
foreach ($username in $usernames)
{
$usertologoff = $sessions | where-object {$_.namesdata.basenames.username -eq $username.username}
Write-Host "Attempting to logoff"$username.username" ..."
$HVServices.Session.Session_LogoffSessionsForced($usertologoff.id)
Start-sleep 3
}
Disconnect-HVServer * -confirm:$false
Copy/paste the script above into a ps1 file. The result will look like this when calling the .ps1 with the arguments in place:

Note that if the user is not actively logged in, you’ll get a some red text for each one that fails as a heads up. Enjoy the script!